Updated January 2022
To perform a large number of tasks, especially custom automated tasks, it is necessary to connect to the Office 365 tenant with Powershell. If you are familiar with Powershell in Exchange then the commands are very similar. Clearly there are additional cmdlets that are relevant to Office 365 and in other articles we can explore those.
As I promised, there are changes here to take into effect the requirement to support MFA accounts. I have edited the article now to take into effect these updates from Microsoft. The link from Microsoft can be found here.
The main function however is to get the connection running in the first place. To do this you need to start up ‘Windows Powershell ISE’ from your desktop and Run As Administrator. The running as administrator is very important as it will not work if you don’t.
The powershell modules to install are listed here. You don’t need all of them if you are just performing simple tasks on Exchange Online perhaps, but it is handy to have them ready in case you do. Installing them is a one-time thing, then you just import them into your session when you want to make use of them.
Install-Module ExchangeOnlineManagement -Repository PSGallery
install-module msonline -Repository PSGallery
install-module azuread -Repository PSGallery
Install-Module Microsoft.Online.SharePoint.PowerShell -Repository PSGallery
Install-Module MicrosoftTeams -Repository PSGallery
To import these modules into your Powershell sessions after you have installed them, type these commands in the Powershell console.
import-module ExchangeOnlineManagement
import-module msonline
import-module azuread
import-module Microsoft.Online.SharePoint.PowerShell
import-module MicrosoftTeams
If this is the very first time you have run this type of script on your machine, you will need to start by executing this command as a ‘one-off’.
Set-ExecutionPolicy RemoteSigned
After this point on your machine you will be able to run any kind of script via the Powershell ISE. Then you can use the following script to perform the connection. This script, as you can see, performs the import-module that I described earlier so you don’t have to do that every time.
Set-ExecutionPolicy RemoteSigned
#Setup Username and Credentials for non-MFA Accounts
$username = “xxxxx@xxxxxx.onmicrosoft.com”
$password = ConvertTo-SecureString “xxxxxx” -AsPlainText -Force
$UserCredential = new-object -typename System.Management.Automation.PSCredential -ArgumentList $username, $password
#Setup Username if an MFA Account
$mfauser = "xxxxx@xxxxxx.onmicrosoft.com"
#Add the SharePoint Admin site name if connecting to SPO.
$spourl = "tenantname-admin.onmicrosoft.com"
#To Connect to Microsoft 365 Exchange Online
#If User is Non-MFA use this connection
Connect-ExchangeOnline -Credential $UserCredential -ShowProgress:$true -showbanner:$false
#If user is MFA use this connection
#Connect-ExchangeOnline -UserPrincipalName <UPN>-ShowProgress:$true -showbanner:$false
#To Connect to Microsoft 365 Users/Licensing Functions
import-module msonline
connect-MsolService -Credential $UserCredential
#To Connect to Azure AD
import-module azuread
connect-AzureAD -Credential $UserCredential
#To Connect to SharePoint Online
import-module Microsoft.Online.SharePoint.PowerShell
Connect-SPOService -Url $spourl -Credential $usercredential
#To Connect to MicrosoftTeams
import-module MicrosoftTeams
Connect-MicrosoftTeams -Credential $usercredential
Replace the items with X’s with the credentials for your tenant. You can also comment out any connections that you don’t need to make. The end result will look like this.
From here you can use normal Exchange style PowerShell to perform functions on Office 365.
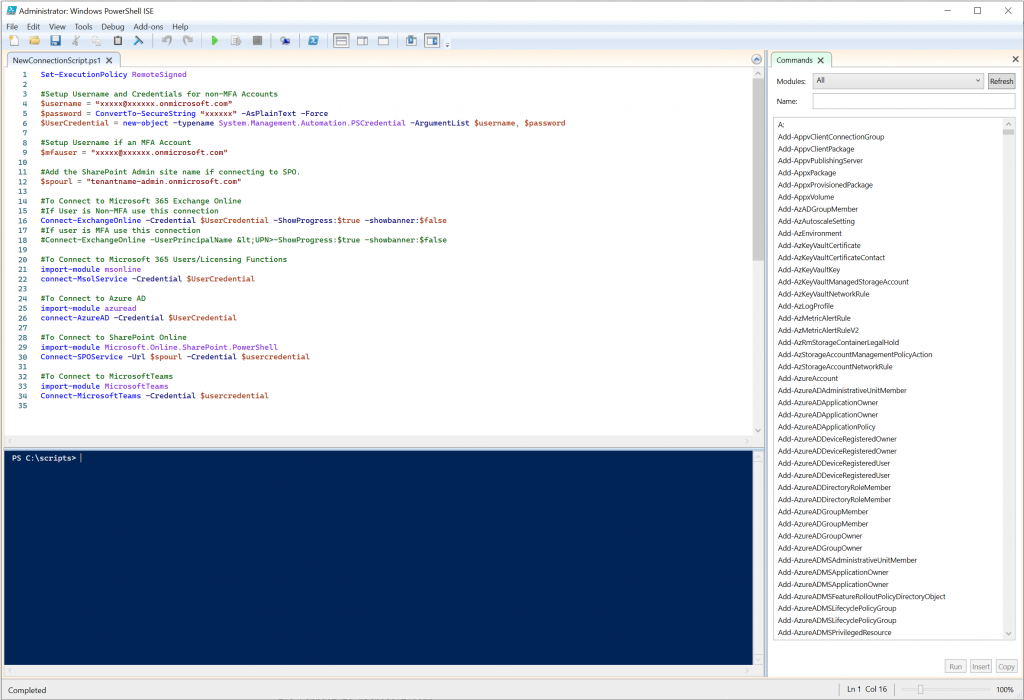
Check Modules Installed
A handy script to check if you have all the right modules installed can be run as a function. Have a look at this. Include it at the top of your script, then just add a ‘CheckPSModules’ command before the main block to ensure that all the modules are installed and ready to go.
function CheckPSModules {
Log-Write "Checking Required PowerShell Modules" -ForegroundColor Green
if ((Get-Module -ListAvailable -Name ExchangeOnlineManagement) -eq $null) {
Log-Write "EXO PowerShell Module Not Found" -ForegroundColor Yellow
$install = Read-Host Do you want to install module? [Y] Yes [N] No
if($install -match "[yY]")
{
Log-Write "Installing EXO PowerShell module" -ForegroundColor Cyan
Install-Module ExchangeOnlineManagement -Repository PSGallery -AllowClobber -Force
}
} else {
Log-Write "EXO PowerShell Module Found" -ForegroundColor Green
}
if ((Get-Module -ListAvailable -Name MSOnline) -eq $null) {
Log-Write "MSOnline PowerShell Module Not Found" -ForegroundColor Yellow
$install = Read-Host Do you want to install module? [Y] Yes [N] No
if($install -match "[yY]")
{
Log-Write "Installing MSOnline PowerShell module" -ForegroundColor Cyan
Install-Module MSOnline -Repository PSGallery -AllowClobber -Force
}
} else {
Log-Write "MSOnline PowerShell Module Found" -ForegroundColor Green
}
if ((Get-Module -ListAvailable -Name AzureAD) -eq $null) {
Log-Write "AzureAD PowerShell Module Not Found" -ForegroundColor Yellow
$install = Read-Host Do you want to install module? [Y] Yes [N] No
if($install -match "[yY]")
{
Log-Write "Installing AzureAD PowerShell module" -ForegroundColor Cyan
Install-Module AzureAD -Repository PSGallery -AllowClobber -Force
}
} else {
Log-Write "AzureAD PowerShell Module Found" -ForegroundColor Green
}
if ((Get-Module -ListAvailable -Name Microsoft.Online.SharePoint.PowerShell) -eq $null) {
Log-Write "SharePoint PowerShell Module Not Found" -ForegroundColor Yellow
$install = Read-Host Do you want to install module? [Y] Yes [N] No
if($install -match "[yY]")
{
Log-Write "Installing SharePoint PowerShell module" -ForegroundColor Cyan
Install-Module Microsoft.Online.SharePoint.PowerShell -Repository PSGallery -AllowClobber -Force
}
} else {
Log-Write "SharePoint PowerShell Module Found" -ForegroundColor Green
}
if ((Get-Module -ListAvailable -Name MicrosoftTeams) -eq $null) {
Log-Write "Teams PowerShell Module Not Found" -ForegroundColor Yellow
$install = Read-Host Do you want to install module? [Y] Yes [N] No
if($install -match "[yY]")
{
Log-Write "Installing Teams PowerShell module" -ForegroundColor Cyan
Install-Module MicrosoftTeams -Repository PSGallery -AllowClobber -Force
}
} else {
Log-Write "Teams PowerShell Module Found" -ForegroundColor Green
}
Log-Write "`nModule Check Completed" -ForegroundColor Green
}